The following script get a list of SharePoint groups on a single site that the user is member of on a single site. You can then either remove the user from all the groups if the user no longer require access, assign the same permissions to another user, or simply display it.
#Connec to SharePoint Online
$TenantAdminURL = "https://tenant-admin.sharepoint.com"
#Connect when the Multifactor Authentication is not enabled
$credentials = Get-Credential
Connect-SPOService -url $TenantAdminURL -Credential $credentials
#Connect using the Multifactor Authentication
Connect-SPOService -url $TenantAdminURL
$searchforuser = "username@sharepoint.run"
$adduser = "new@sharepoint.run"
$removeuser= "retired@sharepoint.run"
#Initialize the array
$SPOGroups = [System.Collections.ArrayList]::new()
$siteurl = "https://tenant.sharepoint.com/sites/sitename"
#Where are getting list of all the groups but without the Shared Links
$Groups = Get-SPOSiteGroup -Site $siteurl | Where {-NOT $_.LoginName.startswith("SharingLinks")}
#Iterating through the Groups in the site to see if the User is member of
ForEach($Group in $Groups)
{
write-host "Current Group:"$Group.LoginName
if($Group.Users -contains $searchforuser){
Wite-host "User Member of Site:" $site.url ",Group:" $Group.LoginName
$SPOGroups.add($Group.LoginName)
}
}
ForEach($SharePointGroup in $groupfound)
{
# Add-SPOUser -Site $siteurl -LoginName $adduser -Group $SharePointGroup
# Remove-SPOUser -Site $siteurl -LoginName $removeuser -Group $SharePointGroup
}
$TenantAdminURL = "https://tenant-admin.sharepoint.com"
This line connect to the SharePoint Online Instance and saves it in a variable called $TenantAdmin. All variables in PowerShell(PS) starts with $ sign.
#Connect when the Multifactor Authentication is not enabled
$credentials = Get-Credential
Connect-SPOService -url $TenantAdminURL -Credential $credentials
#Connect using the Multifactor Authentication
Connect-SPOService -url $TenantAdminURL
If Multifactor authentication is not enabled on your account, You can call “Get-Credential” and a pop up similar to one displayed below will appear where you can provide the userid/password, which they then be saved to variable $credentials, which you can pass to “Connect-SPOService”
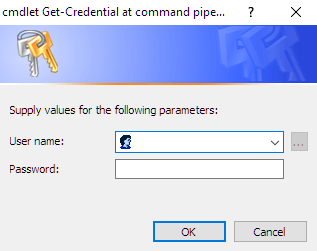
If Multifcator authentication is enabled, then you will be need to be authenticated on the platform itself.
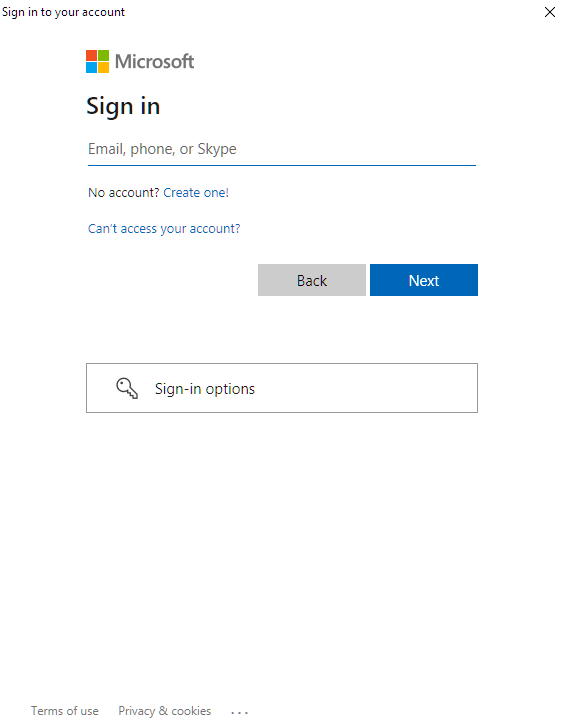
Once authenticatied
$searchforuser = "username@sharepoint.run"
$adduser = "new@sharepoint.run"
$removeuser= "username@sharepoint.run"
You can then replace the “username@sharepoint.run” with the userid that you want to search, additionally if you would like to add user then assign the value to $adduser, and for removing the user from the current groups provide the $removeuser.
#Initialize the array
$SPOGroups = [System.Collections.ArrayList]::new()
We initialize the array, so that we can save the list of all the groups a user is member of, we can then use this array to either remove the user or add another user to same list of SharePoint groups.
$siteurl = "https://tenant.sharepoint.com/sites/sitename"
Provide the site URL of the SharePoint online site that you want to search for a particular user.
#Where are getting list of all the groups but without the Shared Links
$Groups = Get-SPOSiteGroup -Site $siteurl | Where {-NOT $_.LoginName.startswith("SharingLinks")}
We want to get list of All the SharePoint groups, except for the Sharing Links that are individual shared files or folders, shared using Links.
#Iterating through the Groups in the site to see if the User is member of
ForEach($Group in $Groups)
{
write-host "Current Group:"$Group.LoginName
if($Group.Users -contains $searchforuser){
Wite-host "User Member of Site:" $site.url ",Group:" $Group.LoginName
$SPOGroups.add($Group.LoginName)
}
}
We are iterating(ForEach) through all the SharePoint groups($Groups) for one site and checking(-Contains statement) if the user is part of that group. If the user is part of the group, we are then adding the Group to the list in the variable $SPOGroups.
$SPOGroups
To Display the List of the Groups that the User is member of, simply type $SPOGroups in the command prompt
ForEach($SharePointGroup in $groupfound)
{
# Add-SPOUser -Site $siteurl -LoginName $adduser -Group $SharePointGroup
# Remove-SPOUser -Site $siteurl -LoginName $searchforuser -Group $SharePointGroup
}
Additionally, after reviewing, if you would like to remove the user them uncomment the “Remove-SPOUser” line. To Copy the permissions to another User, uncomment the “Add-SPOUser”